|
Home |
Documentation |
Statistics |
Software |
|
Introduction |
Winning a game |
The robot clients |
The communication protocol |
Introduction
The games take place on a server which is waiting for the robot clients to connect.
The communication between the clients and the server is a simple TCP/IP based protocol.
The robot clients can therefore be written in every programming language that
can handle such a communication.
The alien environment on which the robots have to find the goal field,
is a map of hexagonal fields, which can be free-, energy-, start-, or goal-fields.
On these fields can be objects and robots, but only one per field.
Winning a game
Several robots, at the moment 2, 6, 12 or 24, divided into two teams, try to reach the goal field in the alien
environment. The team with the robot that steps on the goal field wins (and ends the game).
The recorded statistics of a game will contain the number of turns until the goal was reached,
the number of robots and which team won.
The robot clients
Each robot has a number of attributes: speed, sight, power, and energy.
Each attribute is represented by a number, e.g. speed=4. The sum of these attributes must be equal to 22.
Speed: Robots can do as many actions per turn as the number of their speed attribute.
Example: a robot with speed=4 can do 4 actions per turn, a robot with speed=10 can do 10 actions per turn.
Actions are either 'move', 'push' or 'idle'. Robots can also 'shout' during their turn, but this does not count
as an action in the 'speed' attribute way.
Sight: Whenever a robot is asked for his next action, the robot is presented a list of fields that form
it's environment. The bigger the sight-number is, the farther the robot can see.
Example: a robot with sight=1 can see the six hexagonal fields that surround it. A robot with sight=2 can see the
six surrounding fields plus the twelve fields with a distance of two.
Power: A robot can only push objects or robots with a weight less or equal to its power. Example:
a robot with power=2 can push an object with weight=2, weight=1 or weight=0. Every robot has a weight of 5.
Energy: Every action of a robot consumes energy. Therefore it is important to reach an energy field before
the energy of an robot reaches 0, because a robot without energy can not move or push something. If a
robot steps on an energy field, the current energy of the robot is restored to its original value. Example:
a robot with energy=10 must reach the next energy field at least with it's 10th action.
The communication protocol
The communication consists of lines of ASCII characters terminated by a newline character ("\n").
A robot must answer within 20 seconds. The server might need more time, especially before the game
is started.
If at any time the server decides that the game has to be stopped without a winner, it will send a deny.
Examples for such situations are: all robots ran out of energy, a robot does not conform to the protocol,
a robot does not answer within some seconds.
DENY \n
The communication is started by the robot client. It sends a line that defines the version of the
communication specification that the robots conforms to, a name that identifies the robot,
the team to which the robot belongs, and how many bots will participate in it's team.
AUTH protocol-version name teamid nbots \n
Currently the protocol-version must be 0, and nbots can be 1, 3, 6, or 12.
'Name' can be freely chosen but must be shorter then 40 characters and must not contain whitespace.
The first 20 characters of the name will show up in the statistics page.
'Teamid' can also freely be chosen, but must also not contain whitespace and must also be shorter
than 40 characters. The server will wait until 'nbots' with the same 'teamid' have connected until it will
start a game with two equal sized teams.
After the authentification, the game server answers with a hello and the goal of the game.
At the moment, this goal is always 'find', but in the future this might change to include
other tasks like 'transport':
HELO FIND \n
The robot client must then tell the server about it's choices for the distribution of 22 points among it's
attributes:
ATTR speed sight power energy \n
After this initialization, the actual game starts with one turn after the other in
random order among the robots. If it is time for a robot to do something, it will receive the following message:
TURN env1 env2 ... envN \n
The number of
env elements is determined by the sight parameter of the robot: In python, this could be
written as
N = sum(n*6 for n in range(sight+1)).
The env elements consist of the following letters:
- f for free field,
- e for energy field,
- s for start field,
- g for goal field,
- o for object,
- r for robot,
- v for void (outside of the map).
The first six elements are the surrounding fields, the next twelve elements start in the same direction as the
first six and are also ordered in the same clockwise direction.
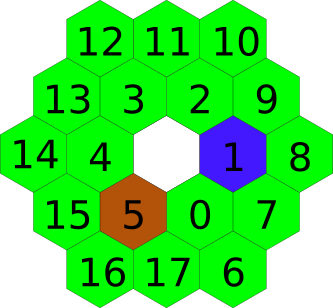
Objects and robots are always above everything
else, so if a robot is standing on an energy field, other robots will see an
r and not an
e.
The robot client can now answer one of four things: that the robot wants to move, to push something, to shout
or to do nothing:
The robot can only move on free fields within the map and only if it's energy is greater than zero. (Note: although
a robot can not move onto a field with a robot or an object, all robots are placed initially on the start fields,
this is therefore the only situation in which several robots can be on the same field.)
Everytime a robot tries to move, it will reduce it's energy by one.
The direction parameter is a number between 0 and 5. Each number identifies a direction. The number also corresponds to the
nth element in the environment list that the robot was presented together with the turn command. So if the robot
received: 'TURN f e f f f o \n', then it can move on the energy field by answering: 'MOVE 1 \n'.
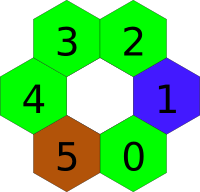
Every move will be one step. If the energy and the speed allow it, the robot will be asked
several times per turn, what it wants to do.
MOVE direction \n
A robot can only push robots and objects that weigh less or equal to it's power and only if it's
energy is greater than zero.
Everytime a robot tries to push, it will reduce it's energy by one. The direction has the same meaning as in
the move command. Note that the robot will not change it's position with a push command.
PUSH direction \n
A robot can shout, even if its energy is zero and shouting does not reduce the energy of a robot.
It does also not reduce the number of moves and pushes a robot can do per turn.
The message will be heard by all robots in the range defined by the range parameter.
The range paramter must be equal to or smaller then 10.
The message must not be longer than 140 characters.
(Note: This is a convenience function to allow the programmers to release many identical robots on a game
without the need to implement a central 'mind'. These simple robots can then locally exchange information
and thereby coordinate their work.)
SHOU range message \n
If a robot is in the defined range, it will receive the following:
LIST message \n
A robot can also decide to do nothing. This does not consume energy but does count as an action. Example:
a robot with speed=2 could answer 'move' and 'idle' and then it's turn would be over.
IDLE \n
If a robot steps onto the goal field, it and all its team members will receive a:
WIN! \n
all robots of the other team will receive a:
LOSE \n
And this ends the game!